คืนค่าการตั้งค่าทั้งหมด
คุณแน่ใจว่าต้องการคืนค่าการตั้งค่าทั้งหมด ?
ลำดับตอนที่ #12 : Java IV - Fundamentals of OOP
Class
Definition and Instantiation
Class
Definitions
The basic steps to create a class is
same as what we learned in Java
III. When nothing is inside a class (see the example below), the class
is a blank design. Using this empty class as a starting point, we can create
instances from scratch.
Creating
Instances
We create an instance from a class as
follows: new ClassName(). Shown in the
figure below, the instance is created based on an empty class, so it doesn't
have any properties or actions yet.
Assigning
Instances to Variables
To use an instance, we assign it to a
variable as follows: ClassType variableName = new
ClassName(). Previously, we specified the data type in front of the
variable name. Now, we specify the class type in front of the instance,
instead.
Creating
Multiple Instances
We can create as many instances as we want from one class. In other words, we can easily mass-produce similar objects based on a design. Instances based on the same class all have the same type of properties and actions. We'll learn about this in detail from the next section.
Instance
Methods
Instance
Fields and Instance Methods
So far, we've learned that instances
have properties and actions. In the programming world, we call properties instance fields and actions
instance methods. We
will learn about them one by one, so you don't have to memorize these terms
now.
Defining
Instance Methods
Let's first learn about instance methods. First of
all, we think of how we want the instance to behave. Since the instance is a
person, let’s give it an action to greet by defining an instance method hello.
Defining
Methods
In a program, a method is used to determine
the behavior of an instance. Such a method is called an instance method, which
we define as follows: public returnValueType
methodName(). Unlike what we learned in Java III, the instance method has no static. We'll learn more about this later on.
Calling
Instance Methods
An instance method belongs to each instance
although we define it inside a class. Therefore, to call an instance method, we
point to an instance (or variable it's assigned to). Like what we learned in
Java III, we use . to point to an instance.
The syntax to call an instance method is as follows: instanceName.methodName().
Instance
Fields
Next, we will learn about the instance field, which is equivalent to the instance's state. We first think of properties that we want an instance to have, and then declare them inside the class. Since the instance is a person, let's give it a property, name.
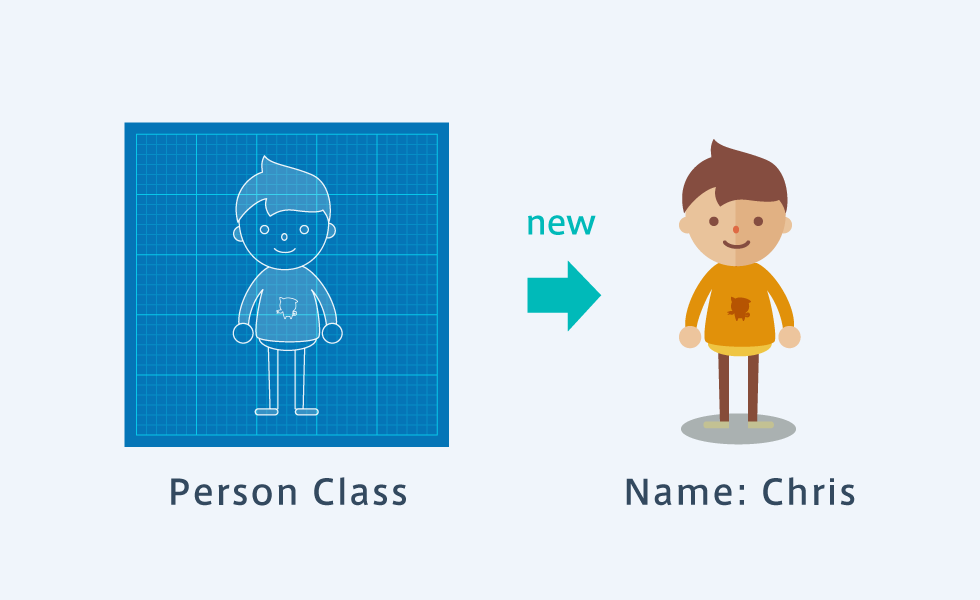
Declaring
Instance Fields
An instance field is simply a variable that stores
information. We declare these variables at the very top of the class body.
Let's make sure to add public in front of
the variable type as follows: public dataType
variableName. We'll lean about the semantics of public
later on.
Creating
Multiple Instance Fields
The instance field also belongs to
each instance. Conceptually, fields are copied to each instance when a new
instance is being created.
Accessing
Instance Fields
In order to access the instance field,
we use . to point to the instance as
follows: instanceName.fieldName(). Instance
fields can be treated just like variables. As shown below, we can assign and
access the value of an instance field.
This
Using
Instances inside Classes
Let’s print, “Hey,
I am ___”, using the name instance field. To do this, we need to access
the value of the name field in the hello method.
This
To access an instance field inside a
method, we use a special variable called this.
We can only use this in the body of a
method. When an instance calls the method, this
is replaced by the instance.
Constructors
Setting
Instance Field Values
In the previous sections, we first
created an instance and then set a value to the name
field. However, this process becomes troublesome as the number of instance
fields increases. There is an easy way to set a value to each instance field.
We'll learn about this trick through the next two exercises.
Constructors
A class has a constructor. A constructor is a special method that is called
automatically when we create an instance using new.
Defining
the Constructor
We need to stick with the following
two rules to define the constructor since it’s a special method: (1) give the
constructor the same name as its class; and (2) do not write return or void.
If we follow these rules, we can successfully define the constructor.
Constructor
Example
For example, we can write a program to
call the constructor when we create an instance using new
(see below). We need a few more steps to easily set a value to each instance
field. But for now, let's just practice using the constructor.
Setting
Field Values
Let's use the constructor to ease the
hassle of setting a value to each instance field. We'll first create a constructor
that is automatically executed every time we create an instance using new. Then, we will set values to the instance
fields inside the constructor.
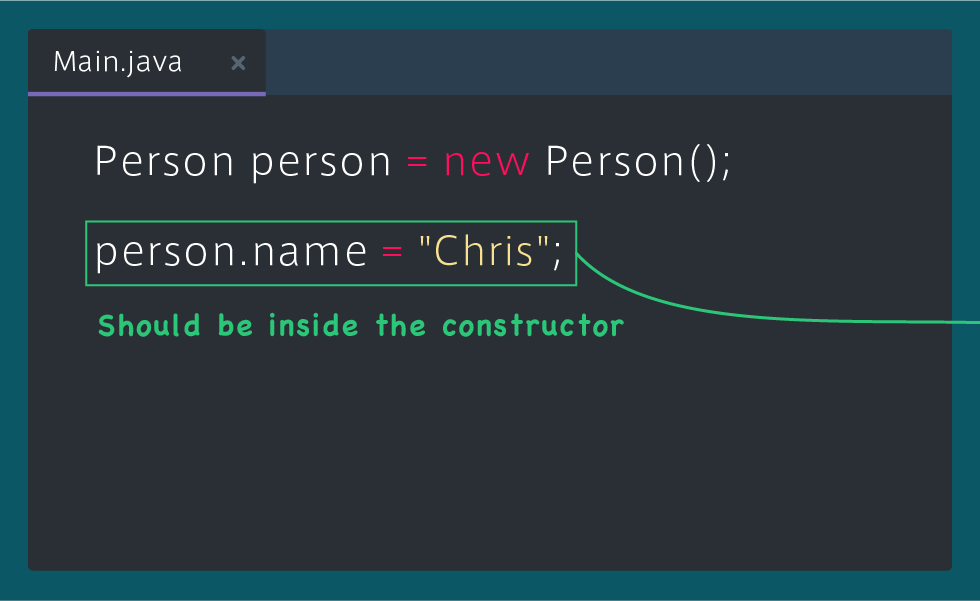
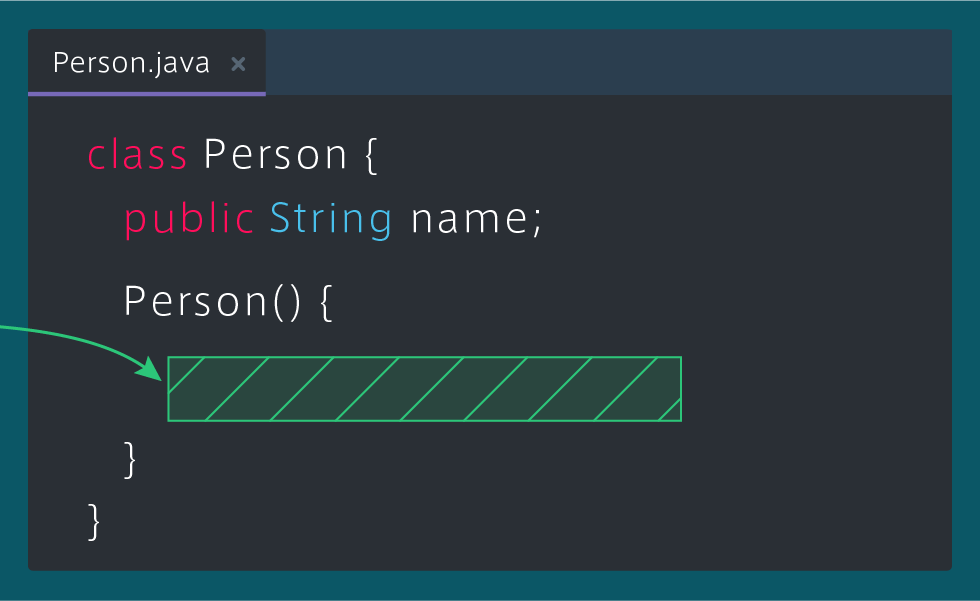
Passing
Information to the Constructor
When we create an instance using new, we can pass arguments to the () of new ClassName().
Then, the arguments are passed to the constructor to be called immediately
after. Using this, we can pass information to the constructor.
Setting
Fields using the Constructor
When we create a new instance, we
first pass a value to the argument to be assigned to the instance field. This
will get passed to the parameters of the constructor, where you can set the
instance fields.
ความคิดเห็น